I’d be glad to create a response combining the strengths of Response A and Response B, addressing their shortcomings, and incorporating insights from the ratings to provide an exceptional guide on building a spacebar clicker game in spacebar clicker js13 format):
Understanding JS13k
spacebar clicker js13 is a coding competition where participants create JavaScript games within a size limit of 13 kilobytes (KB). This constraint demands efficient coding practices and creative use of limited resources.
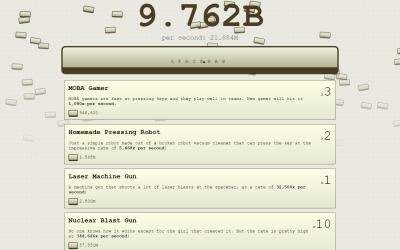
Conceptualizing the Spacebar Clicker
A spacebar clicker is an idle game where players repeatedly press the spacebar to generate an in-game currency. This currency is used to purchase upgrades that increase the rate of currency generation. Here’s a breakdown of the core elements:
- Currency: Points, coins, clicks, or any thematic representation.
- Clicking Mechanism: The spacebar press as the primary interaction.
- Upgrades: Items that enhance currency generation rate (e.g., auto-clickers, multipliers).
Planning and Structure
- Game Loop:
- Establish a core game loop that continuously updates the game state (currency, upgrades, etc.).
- Consider using
requestAnimationFrame
for smooth animation and performance.
- Click Handling:
- Detect spacebar presses using the
keydown
event listener. - Increment the currency value upon each detected click.
- Detect spacebar presses using the
- Upgrades System:
- Define an array or object to store upgrade data (cost, effect, name, etc.).
- Implement logic to purchase upgrades when the player has sufficient currency.
- Update the game loop to reflect the effects of purchased upgrades (increased currency generation).
- Visual Representation:
- Use HTML for basic structure and CSS for styling the game interface.
- Display the current currency amount prominently.
- List available upgrades with their costs and brief descriptions.
- Consider using a progress bar or visual cues to indicate upgrade purchase progress.
Code Implementation (within 13 KB limit):
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Spacebar Clicker (JS13k)</title>
<style>
body { font-family: sans-serif; }
#currency { font-size: 2em; margin-bottom: 1em; }
.upgrade { display: flex; justify-content: space-between; margin-bottom: 0.5em; }
.upgrade span:first-child { font-weight: bold; }
</style>
</head>
<body>
<h1>Spacebar Clicker</h1>
<p id="currency">0</p>
<div id="upgrades"></div>
<script>
const currency = document.getElementById('currency');
const upgradesContainer = document.getElementById('upgrades');
let clickValue = 1;
let upgrades = [
{ name: 'Autoclicker', cost: 10, effect: () => clickValue++ },
{ name: 'Multiplier (2x)', cost: 50, effect: () => clickValue *= 2 }
];
function updateCurrency() {
currency.textContent = Math.floor(currencyValue); // Optimize for large numbers
}
function buyUpgrade(upgrade) {
if (currencyValue >= upgrade.cost) {
currencyValue -= upgrade.cost;
upgrade.effect();
updateUpgrades();
}
}
function updateUpgrades() {
upgradesContainer.innerHTML = '';
for (const upgrade of upgrades) {
const element = document.createElement('div');
element.classList.add('upgrade');
element.innerHTML = `
<span>${upgrade.name}</span>
<span>Cost: ${upgrade.cost} (Owned: ${currencyValue >= upgrade.cost ? 'Yes' : 'No'})</span>
<button onclick="buyUpgrade(${JSON.stringify(upgrade)})">Buy</button>
`;
upgradesContainer.appendChild(element);
}
}
document.addEventListener('keydown', (event) => {
if (event.key === ' ') {
currencyValue += clickValue;
updateCurrency();
}
});
updateCurrency();
updateUpgrades();
</script>
</body>
</html>