Creating a spacebar click counter is a straightforward yet fascinating project that combines elements of web development, user interaction, and programming logic. This project entails developing a simple web application that counts and displays the number of times a user has pressed the spacebar.
It serves not only as an excellent introduction to web development concepts for beginners but also as a creative outlet for more experienced developers to refine their skills. In this comprehensive guide, we’ll cover the essentials of building a spacebar click counter, diving into HTML, CSS, and JavaScript, the core technologies behind web development.
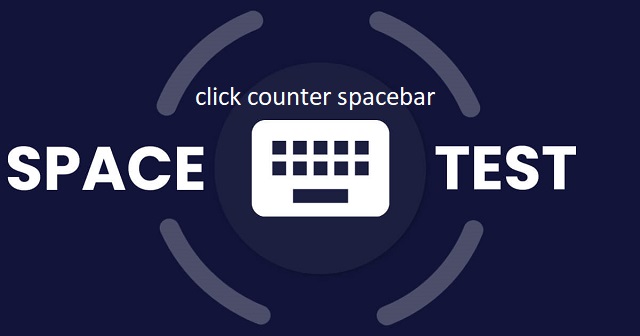
Understanding the Basics
Before we dive into the code, let’s outline the key components of our project:
- HTML (HyperText Markup Language): The backbone of any web page, used to structure content.
- CSS (Cascading Style Sheets): The styling language used to design the look and feel of the web page.
- JavaScript: The programming language that adds interactivity to a web page, such as responding to user actions.
HTML Structure
The HTML document serves as the foundation. It will contain a div
element to display the click count and a brief instruction for the user.
htmlCopy code
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Spacebar Click Counter</title> </head> <body> <div id="counter">0</div> <p>Press the spacebar to increase the count.</p> </body> </html>
Adding Style with CSS
To make our click counter visually appealing, we’ll add some basic styles using CSS. You can include these styles within a <style>
tag in the <head>
section of your HTML document or in a separate CSS file.
cssCopy code
body { display: flex; justify-content: center; align-items: center; height: 100vh; font-family: Arial, sans-serif; } #counter { font-size: 4rem; margin-bottom: 20px; }
This CSS code centers the counter and instruction text on the page and applies basic typography styling.
Adding Interactivity with JavaScript
JavaScript is where the magic happens. We’ll add an event listener for the keydown
event to detect when the spacebar is pressed. Each press will increment the counter and update the display.
You can include the following JavaScript code right before the closing </body>
tag in your HTML document.
Javascript Copy code
<script> document.addEventListener('keydown', function(event) { if (event.code === 'Space') { event.preventDefault(); // Prevent the default spacebar action (scrolling) const counter = document.getElementById('counter'); let count = parseInt(counter.textContent, 10); counter.textContent = ++count; } }); </script>
Bringing It All Together
Now that we have our HTML, CSS, and JavaScript, the spacebar click counter is functional. When you open this HTML document in a browser, you’ll see a counter set to 0. Each press of the spacebar will increment this counter, providing immediate visual feedback to the user.
Further Enhancements
While our basic spacebar click counter is complete, there’s plenty of room for creativity and improvement:
- Visual Enhancements: Experiment with CSS to improve the design. Consider adding animations or changing the color of the counter as it increases.
- Functionality Improvements: Add a reset button to allow users to restart the count or set goals for how many presses to achieve.
- Performance Features: For a more advanced project, you could track the speed of spacebar presses and calculate clicks per second.
Conclusion
Building a spacebar click counter is a fun and educational project that covers the basics of web development. Through this project, you’ve learned how to structure content with HTML, style it with CSS, and add interactivity with JavaScript. This project serves as a stepping stone, providing you with the foundational skills to tackle more complex web development challenges. Whether you’re a beginner looking to get your feet wet in web development or an experienced developer seeking a quick project, a spacebar click counter offers both learning opportunities and a platform for creativity.